Java Gui Read Data File Into a Database
File choosers provide a GUI for navigating the file organisation, and and so either choosing a file or directory from a list, or inbound the name of a file or directory. To display a file chooser, you usually use the JFileChooser
API to show a modal dialog containing the file chooser. Another mode to nowadays a file chooser is to add an case of JFileChooser
to a container.
Notation:
If y'all intend to distribute your program as a sandbox Java Web Kickoff awarding, then instead of using the JFileChooser
API you should use the file services provided by the JNLP API. These services — FileOpenService
and FileSaveService
— not simply provide back up for choosing files in a restricted surroundings, but also take care of actually opening and saving them. An instance of using these services is in JWSFileChooserDemo. Documentation for using the JNLP API tin exist found in the Java Spider web Start lesson.
Click the Launch button to run JWSFileChooserDemo using Java™ Spider web Start (download JDK seven or subsequently). Alternatively, to compile and run the example yourself, consult the example index.
When working with the JWSFileChooserDemo
case, be careful not to lose files that you need. Whenever y'all click the save button and select an existing file, this demo brings upwardly the File Exists dialog box with a asking to replace the file. Accepting the request overwrites the file.
The rest of this department discusses how to use the JFileChooser
API. A JFileChooser
object only presents the GUI for choosing files. Your plan is responsible for doing something with the chosen file, such as opening or saving it. Refer to Bones I/O for information on how to read and write files.
The JFileChooser
API makes it easy to bring up open up and save dialogs. The type of look and feel determines what these standard dialogs look like and how they differ. In the Java await and feel, the save dialog looks the same as the open dialog, except for the title on the dialog'due south window and the text on the button that approves the operation. Here is a picture of a standard open dialog in the Java await and experience:

Here is a picture of an application chosen FileChooserDemo
that brings up an open up dialog and a salve dialog.
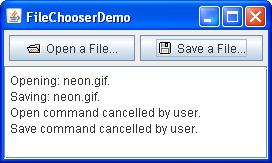
Try this:
- Compile and run the example, consult the example index.
- Click the Open a File push. Navigate around the file chooser, choose a file, and click the dialog's Open up button.
- Use the Save a File button to bring upwardly a salve dialog. Endeavour to use all of the controls on the file chooser.
- In the source file
FileChooserDemo.coffee
, change the file selection mode to directories-just mode. (Search forDIRECTORIES_ONLY
and uncomment the line that contains information technology.) Then compile and run the example again. You lot will only exist able to run across and select directories, non ordinary files.
Bringing up a standard open dialog requires only two lines of lawmaking:
//Create a file chooser final JFileChooser fc = new JFileChooser(); ... //In response to a push button click: int returnVal = fc.showOpenDialog(aComponent);
The argument to the showOpenDialog
method specifies the parent component for the dialog. The parent component affects the position of the dialog and the frame that the dialog depends on. For instance, the Java look and feel places the dialog straight over the parent component. If the parent component is in a frame, then the dialog is dependent on that frame. This dialog disappears when the frame is minimized and reappears when the frame is maximized.
Past default, a file chooser that has non been shown before displays all files in the user'south dwelling house directory. You can specify the file chooser's initial directory by using one of JFileChooser
'south other constructors, or you can prepare the directory with the setCurrentDirectory
method.
The telephone call to showOpenDialog
appears in the actionPerformed
method of the Open up a File push button's action listener:
public void actionPerformed(ActionEvent e) { //Handle open button action. if (eastward.getSource() == openButton) { int returnVal = fc.showOpenDialog(FileChooserDemo.this); if (returnVal == JFileChooser.APPROVE_OPTION) { File file = fc.getSelectedFile(); //This is where a real application would open the file. log.append("Opening: " + file.getName() + "." + newline); } else { log.append("Open command cancelled past user." + newline); } } ... }
The showXxxDialog
methods return an integer that indicates whether the user selected a file. Depending on how y'all use a file chooser, it is ofttimes sufficient to check whether the return value is APPROVE_OPTION
then not to modify any other value. To go the chosen file (or directory, if you ready the file chooser to let directory selections), call the getSelectedFile
method on the file chooser. This method returns an example of File
.
The case obtains the name of the file and uses information technology in the log message. You can call other methods on the File
object, such as getPath
, isDirectory
, or exists
to obtain information near the file. You tin can as well phone call other methods such equally delete
and rename
to modify the file in some style. Of course, you might also want to open or save the file by using one of the reader or writer classes provided past the Coffee platform. See Basic I/O for information most using readers and writers to read and write information to the file system.
The case program uses the same case of the JFileChooser
course to display a standard salve dialog. This time the program calls showSaveDialog
:
int returnVal = fc.showSaveDialog(FileChooserDemo.this);
By using the same file chooser case to brandish its open and salve dialogs, the program reaps the following benefits:
- The chooser remembers the current directory between uses, so the open up and salvage versions automatically share the same current directory.
- You have to customize only one file chooser, and the customizations use to both the open and salve versions.
Finally, the example program has commented-out lines of lawmaking that let you alter the file pick mode. For example, the following line of code makes the file chooser able to select only directories, and not files:
fc.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
Another possible selection mode is FILES_AND_DIRECTORIES
. The default is FILES_ONLY
. The post-obit picture show shows an open dialog with the file selection mode gear up to DIRECTORIES_ONLY
. Notation that, in the Java look and feel at least, simply directories are visible — not files.

If yous want to create a file chooser for a task other than opening or saving, or if you lot want to customize the file chooser, keep reading. We will discuss the following topics:
- Another Example: FileChooserDemo2
- Using a File Chooser for a Custom Chore
- Filtering the List of Files
- Customizing the File View
- Providing an Accessory Component
- The File Chooser API
- Examples that Use File Choosers
Another Example: FileChooserDemo2
Permit us wait at FileChooserDemo2
case, a modified version of the previous demo program that uses more of the JFileChooser
API. This instance uses a file chooser that has been customized in several ways. Similar the original instance, the user invokes a file chooser with the push of a button. Here is a picture of the file chooser:

Equally the figure shows, this file chooser has been customized for a special task (Attach), provides a user-choosable file filter (But Images), uses a special file view for image files, and has an accessory component that displays a thumbnail sketch of the currently selected image file.
The remainder of this section shows you the lawmaking that creates and customizes this file chooser. Encounter the example index for links to all the files required past this case.
Using a File Chooser for a Custom Chore
As you accept seen, the JFileChooser
class provides the showOpenDialog
method for displaying an open dialog and the showSaveDialog
method for displaying a salvage dialog.
The course has another method, showDialog
, for displaying a file chooser for a custom chore in a dialog. In the Java look and feel, the only deviation between this dialog and the other file chooser dialogs is the title on the dialog window and the label on the corroborate button. Here is the code from FileChooserDemo2
that brings up the file chooser dialog for the Adhere task:
JFileChooser fc = new JFileChooser(); int returnVal = fc.showDialog(FileChooserDemo2.this, "Attach");
The get-go argument to the showDialog
method is the parent component for the dialog. The 2d statement is a Cord
object that provides both the title for the dialog window and the label for the approve button.
Once once again, the file chooser doesn't exercise annihilation with the selected file. The program is responsible for implementing the custom task for which the file chooser was created.
Filtering the List of Files
By default, a file chooser displays all of the files and directories that it detects, except for hidden files. A plan tin can apply one or more than file filters to a file chooser so that the chooser shows only some files. The file chooser calls the filter's accept
method for each file to determine whether information technology should be displayed. A file filter accepts or rejects a file based on criteria such every bit file type, size, ownership, and so on. Filters impact the list of files displayed by the file chooser. The user can enter the name of any file even if information technology is not displayed.
JFileChooser
supports iii different kinds of filtering. The filters are checked in the order listed here. For example, an application-controlled filter sees just those files accustomed by the built-in filtering.
- Built-in filtering
- Filtering is prepare through specific method calls on a file chooser. Currently the only built-in filter available is for subconscious files, such as those whose names begin with period (.) on UNIX systems. By default, hidden files are not shown. Call
setFileHidingEnabled(simulated)
to show hidden files. - Application-controlled filtering
- The application determines which files are shown. Create a custom subclass of
FileFilter
, instantiate it, and use the instance as an argument to thesetFileFilter
method. The installed filter is displayed on the listing of user-choosable filters. The file chooser shows merely those files that the filter accepts. - User-choosable filtering
- The file chooser GUI provides a list of filters that the user can choose from. When the user chooses a filter, the file chooser shows just those files accustomed by that filter.
FileChooserDemo2
adds a custom file filter to the listing of user-choosable filters:fc.addChoosableFileFilter(new ImageFilter());
fc.setAcceptAllFileFilterUsed(false);
ImageFilter.java
and is a subclass ofFileFilter
. TheImageFilter
class implements thegetDescription
method to render "Only Images" — a string to put in the list of user-choosable filters.ImageFilter
also implements theaccept
method so that it accepts all directories and any file that has a.png
,.jpg
,.jpeg
,.gif
,.tif
, or.tiff
filename extension.public boolean accept(File f) { if (f.isDirectory()) { return true; } String extension = Utils.getExtension(f); if (extension != nothing) { if (extension.equals(Utils.tiff) || extension.equals(Utils.tif) || extension.equals(Utils.gif) || extension.equals(Utils.jpeg) || extension.equals(Utils.jpg) || extension.equals(Utils.png)) { return true; } else { return false; } } return false; }
The preceding code sample uses the
getExtension
method and several string constants fromUtils.java
, shown here:public grade Utils { public final static String jpeg = "jpeg"; public terminal static Cord jpg = "jpg"; public terminal static String gif = "gif"; public final static String tiff = "tiff"; public final static String tif = "tif"; public final static String png = "png"; /* * Get the extension of a file. */ public static String getExtension(File f) { Cord ext = null; String s = f.getName(); int i = s.lastIndexOf('.'); if (i > 0 && i < due south.length() - 1) { ext = s.substring(i+1).toLowerCase(); } render ext; } }
Customizing the File View
In the Coffee look and feel, the chooser's list shows each file's proper noun and displays a small-scale icon that represents whether the file is a true file or a directory. You can customize this file view past creating a custom subclass of FileView
and using an instance of the course as an argument to the setFileView
method. The example uses an instance of a custom course, implemented in ImageFileView.java
, as the file chooser's file view.
fc.setFileView(new ImageFileView());
The ImageFileView
class shows a different icon for each type of image accepted past the image filter described previously.
The ImageFileView
class overrides the 5 abstract methods divers in the FileView
as follows.
-
String getTypeDescription(File f)
- Returns a clarification of the file type. Here is
ImageFileView
's implementation of this method:public String getTypeDescription(File f) { String extension = Utils.getExtension(f); String type = zilch; if (extension != null) { if (extension.equals(Utils.jpeg) || extension.equals(Utils.jpg)) { type = "JPEG Image"; } else if (extension.equals(Utils.gif)){ type = "GIF Image"; } else if (extension.equals(Utils.tiff) || extension.equals(Utils.tif)) { blazon = "TIFF Paradigm"; } else if (extension.equals(Utils.png)){ type = "PNG Image"; } } render blazon; }
-
Icon getIcon(File f)
- Returns an icon representing the file or its type. Here is
ImageFileView
's implementation of this method:public Icon getIcon(File f) { String extension = Utils.getExtension(f); Icon icon = null; if (extension != null) { if (extension.equals(Utils.jpeg) || extension.equals(Utils.jpg)) { icon = jpgIcon; } else if (extension.equals(Utils.gif)) { icon = gifIcon; } else if (extension.equals(Utils.tiff) || extension.equals(Utils.tif)) { icon = tiffIcon; } else if (extension.equals(Utils.png)) { icon = pngIcon; } } return icon; }
-
String getName(File f)
- Returns the name of the file. Most implementations of this method should return
aught
to indicate that the look and feel should effigy it out. Another common implementation returnsf.getName()
. -
Cord getDescription(File f)
- Returns a clarification of the file. The intent is to describe individual files more than specifically. A common implementation of this method returns
nothing
to indicate that the look and feel should figure it out. -
Boolean isTraversable(File f)
- Returns whether a directory is traversable. About implementations of this method should return
null
to indicate that the look and feel should figure information technology out. Some applications might want to foreclose users from descending into a sure blazon of directory because information technology represents a chemical compound document. TheisTraversable
method should never returntrue
for a non-directory.
Providing an Accessory Component
The customized file chooser in FileChooserDemo2
has an accessory component. If the currently selected item is a PNG, JPEG, TIFF, or GIF paradigm, the accessory component displays a thumbnail sketch of the paradigm. Otherwise, the accessory component is empty. Aside from a previewer, probably the most common use for the accessory component is a console with more controls on it such as cheque boxes that toggle between features.
The example calls the setAccessory
method to establish an instance of the ImagePreview
form, implemented in ImagePreview.java
, as the chooser'due south accessory component:
fc.setAccessory(new ImagePreview(fc));
Any object that inherits from the JComponent
class can be an accessory component. The component should have a preferred size that looks good in the file chooser.
The file chooser fires a property change result when the user selects an particular in the list. A program with an accessory component must register to receive these events to update the accessory component whenever the selection changes. In the instance, the ImagePreview
object itself registers for these events. This keeps all the code related to the accessory component together in one class.
Here is the example'southward implementation of the propertyChange
method, which is the method called when a property change event is fired:
//where fellow member variables are alleged File file = null; ... public void propertyChange(PropertyChangeEvent due east) { boolean update = false; String prop = east.getPropertyName(); //If the directory inverse, don't testify an image. if (JFileChooser.DIRECTORY_CHANGED_PROPERTY.equals(prop)) { file = nix; update = truthful; //If a file became selected, discover out which ane. } else if (JFileChooser.SELECTED_FILE_CHANGED_PROPERTY.equals(prop)) { file = (File) due east.getNewValue(); update = true; } //Update the preview accordingly. if (update) { thumbnail = aught; if (isShowing()) { loadImage(); repaint(); } } }
If SELECTED_FILE_CHANGED_PROPERTY
is the property that inverse, this method obtains a File
object from the file chooser. The loadImage
and repaint
methods use the File
object to load the image and repaint the accessory component.
The File Chooser API
The API for using file choosers falls into these categories:
- Creating and Showing the File Chooser
- Selecting Files and Directories
- Navigating the File Chooser's List
- Customizing the File Chooser
Method or Constructor | Purpose |
---|---|
JFileChooser() JFileChooser(File) JFileChooser(Cord) | Creates a file chooser case. The File and String arguments, when nowadays, provide the initial directory. |
int showOpenDialog(Component) int showSaveDialog(Component) int showDialog(Component, String) | Shows a modal dialog containing the file chooser. These methods return APPROVE_OPTION if the user canonical the operation and CANCEL_OPTION if the user cancelled it. Another possible return value is ERROR_OPTION , which means an unanticipated error occurred. |
Method | Purpose |
---|---|
void setSelectedFile(File) File getSelectedFile() | Sets or obtains the currently selected file or (if directory selection has been enabled) directory. |
void setSelectedFiles(File[]) File[] getSelectedFiles() | Sets or obtains the currently selected files if the file chooser is gear up to allow multiple selection. |
void setFileSelectionMode(int) void getFileSelectionMode() boolean isDirectorySelectionEnabled() boolean isFileSelectionEnabled() | Sets or obtains the file selection mode. Acceptable values are FILES_ONLY (the default), DIRECTORIES_ONLY , and FILES_AND_DIRECTORIES .Interprets whether directories or files are selectable according to the current selection style. |
void setMultiSelectionEnabled(boolean) boolean isMultiSelectionEnabled() | Sets or interprets whether multiple files can be selected at in one case. By default, a user can choose only ane file. |
void setAcceptAllFileFilterUsed(boolean) boolean isAcceptAllFileFilterUsed() | Sets or obtains whether the AcceptAll file filter is used as an allowable choice in the choosable filter list; the default value is truthful . |
Dialog createDialog(Component) | Given a parent component, creates and returns a new dialog that contains this file chooser, is dependent on the parent's frame, and is centered over the parent. |
Method | Purpose |
---|---|
void ensureFileIsVisible(File) | Scrolls the file chooser's list such that the indicated file is visible. |
void setCurrentDirectory(File) File getCurrentDirectory() | Sets or obtains the directory whose files are displayed in the file chooser's list. |
void changeToParentDirectory() | Changes the listing to display the current directory's parent. |
void rescanCurrentDirectory() | Checks the file system and updates the chooser's list. |
void setDragEnabled(boolean) boolean getDragEnabled() | Sets or obtains the holding that determines whether automatic drag handling is enabled. See Drag and Drop and Data Transfer for more details. |
Method | Purpose |
---|---|
void setAccessory(javax.swing.JComponent) JComponent getAccessory() | Sets or obtains the file chooser's accompaniment component. |
void setFileFilter(FileFilter) FileFilter getFileFilter() | Sets or obtains the file chooser'due south master file filter. |
void setFileView(FileView) FileView getFileView() | Sets or obtains the chooser's file view. |
FileFilter[] getChoosableFileFilters() void addChoosableFileFilter(FileFilter) boolean removeChoosableFileFilter(FileFilter) void resetChoosableFileFilters() FileFilter getAcceptAllFileFilter() | Sets, obtains, or modifies the list of user-choosable file filters. |
void setFileHidingEnabled(boolean) boolean isFileHidingEnabled() | Sets or obtains whether hidden files are displayed. |
void setControlButtonsAreShown(boolean) boolean getControlButtonsAreShown() | Sets or obtains the property that indicates whether the Approve and Cancel buttons are shown in the file chooser. This property is true by default. |
Examples That Use File Choosers
This table shows the examples that use file choosers and points to where those examples are described.
Example | Where Described | Notes |
---|---|---|
FileChooserDemo | This section | Displays an open dialog and a salvage dialog. |
FileChooserDemo2 | This section | Uses a file chooser with custom filtering, a custom file view, and an accompaniment component. |
JWSFileChooserDemo | This section | Uses the JNLP API to open and relieve files. |
Java Gui Read Data File Into a Database
Source: https://docs.oracle.com/javase/tutorial/uiswing/components/filechooser.html